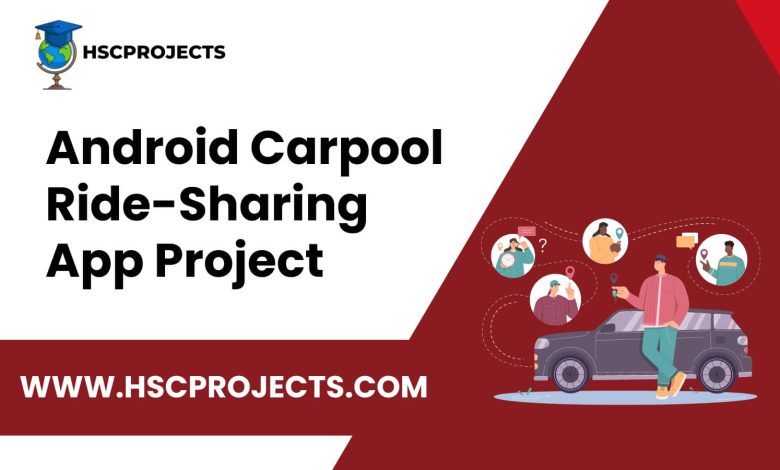
Android Carpool Ride-Sharing App Project
Introduction
Traffic congestion is a growing concern in cities worldwide, and India is no exception. With the rise in population and the number of private vehicles, the need for alternative commuting options has never been greater. Enter the Android carpool ride sharing app—a solution that not only eases the burden on the roads but also offers a more sustainable way to travel.
Why Carpooling?
Carpooling is an eco-friendly and cost-effective way to commute. By sharing a ride with others, you can significantly reduce your carbon footprint and save on fuel costs. However, the traditional method of finding carpool partners can be cumbersome and time-consuming. This is where carpool apps come into play, making it easier for users to offer a ride or find one.

Features of Android Carpool Ride Sharing Apps
- User Verification: To ensure safety, users must undergo a verification process administered by the app’s admin. Only verified users can offer or find rides.
- Offer a Ride: Users can list their cars and set a per-km charge for passengers. They can specify the source, destination, date, and time for the ride.
- Find a Ride: Users can search for rides that match their criteria, such as location, date, and time. Once a match is found, they can request to join the ride.
- Payment System: The app allows users to settle payments either through cash or a built-in payment gateway.
Advantages
- Ease of Use: The user-friendly interface makes it simple to offer or find a ride.
- Cost-Effective: Sharing rides splits the cost, making it a more affordable option.
- Eco-Friendly: Fewer cars on the road mean less pollution and a smaller carbon footprint.
Limitations
- Input Accuracy: The system relies on accurate information from the users. Incorrect inputs can lead to mismatched rides or other issues.
Conclusion
Android carpool ride sharing apps are a boon for urban commuters in India. They offer a convenient, cost-effective, and eco-friendly way to navigate the challenges of daily transportation. So, the next time you’re stuck in traffic, consider using a carpool app to make your journey a little easier.
Sample Code
# Pseudo-code for a simplified Carpool Ride Sharing App
# Database tables
users = {} # user_id -> {name, email, is_verified}
rides = {} # ride_id -> {source, destination, date, time, driver_id, passenger_ids}
# Function to add a new user
def add_user(name, email):
user_id = len(users) + 1
users[user_id] = {'name': name, 'email': email, 'is_verified': False}
return user_id
# Function to verify a user
def verify_user(user_id):
if user_id in users:
users[user_id]['is_verified'] = True
# Function to offer a ride
def offer_ride(user_id, source, destination, date, time):
if users[user_id]['is_verified']:
ride_id = len(rides) + 1
rides[ride_id] = {'source': source, 'destination': destination, 'date': date, 'time': time, 'driver_id': user_id, 'passenger_ids': []}
return ride_id
else:
return "User not verified"
# Function to find a ride
def find_ride(source, destination, date, time):
available_rides = []
for ride_id, ride in rides.items():
if ride['source'] == source and ride['destination'] == destination and ride['date'] == date and ride['time'] == time:
available_rides.append(ride_id)
return available_rides
# Function to join a ride
def join_ride(user_id, ride_id):
if users[user_id]['is_verified']:
if ride_id in rides:
rides[ride_id]['passenger_ids'].append(user_id)
return "Successfully joined the ride"
else:
return "Ride not found"
else:
return "User not verified"
# Function to start a ride (by the driver)
def start_ride(ride_id):
if ride_id in rides:
# Logic to start the ride
return "Ride started"
else:
return "Ride not found"
# Function to end a ride (by the driver)
def end_ride(ride_id):
if ride_id in rides:
# Logic to end the ride
return "Ride ended"
else:
return "Ride not found"
# Function to make a payment (by the passenger)
def make_payment(user_id, ride_id, amount):
if user_id in rides[ride_id]['passenger_ids']:
# Logic to make the payment
return "Payment successful"
else:
return "User not part of the ride"
This is a very basic example and doesn’t cover many features like error handling, real-time tracking, or payment gateway integration. However, it should give you a starting point for developing your own carpool ride-sharing app.
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Android Carpool Ride-Sharing App Project PDF
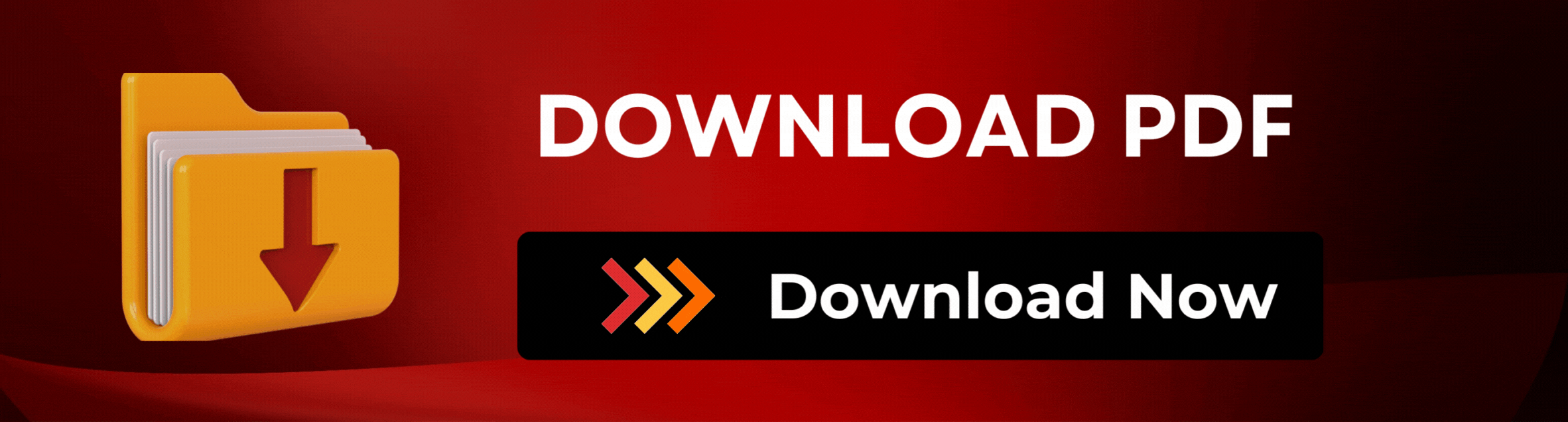