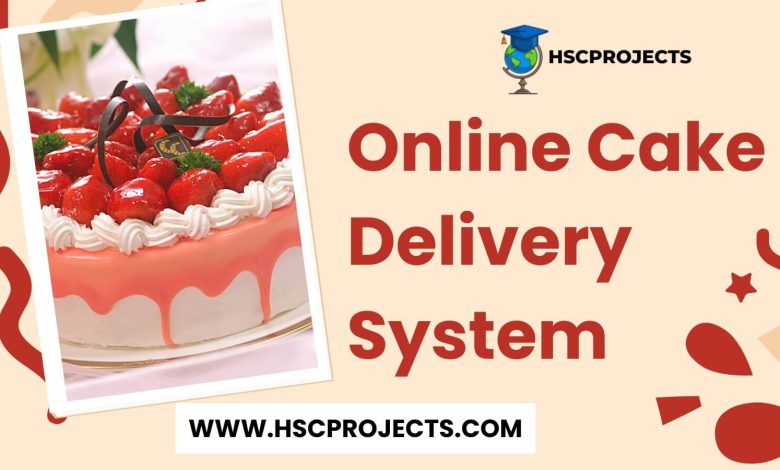
Online Cake Delivery System
Introduction
In today’s fast-paced world, who has the time to visit a cake shop physically? Our Online Cake Delivery System is here to revolutionize your cake shopping experience. Whether you’re in Noida, Denmark, Amritsar, or Goa, our platform offers a wide range of cakes and bakery products that you can order from the comfort of your home.
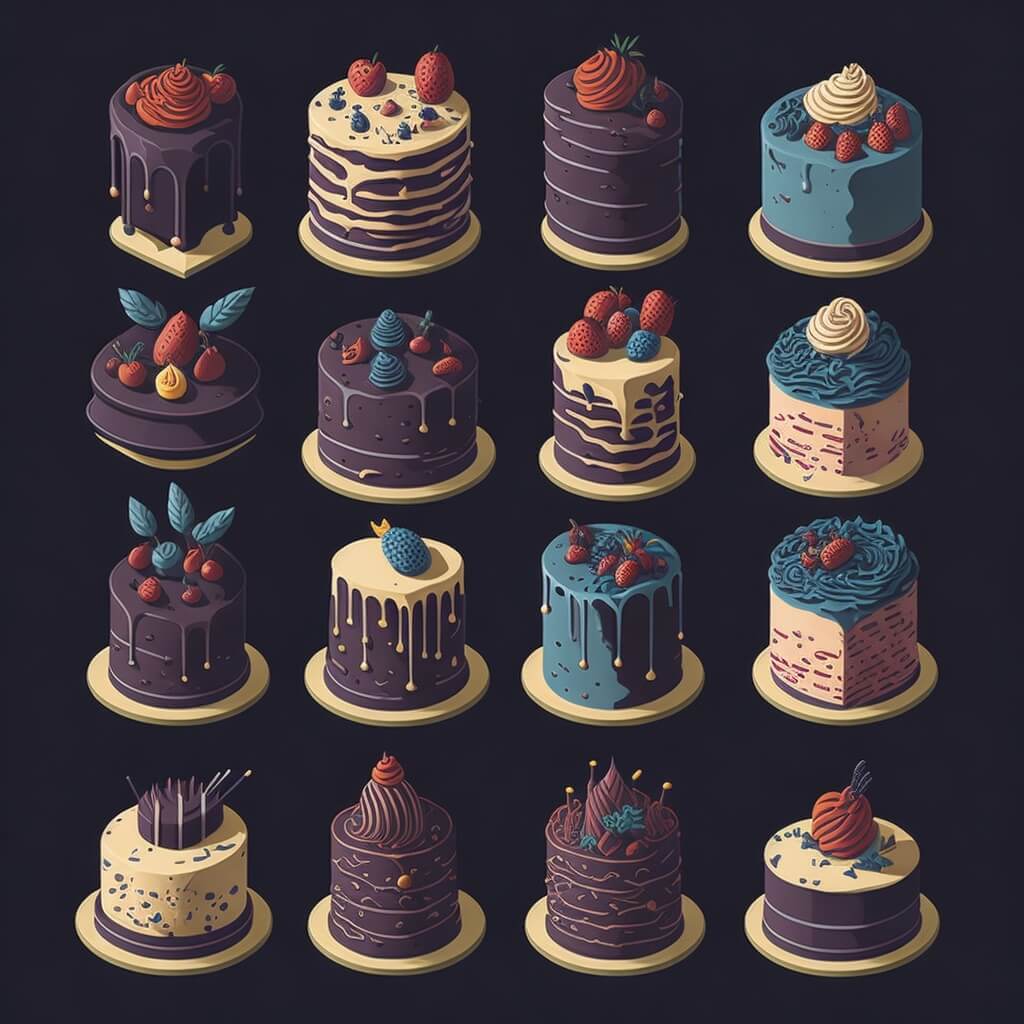
Features
Wide Range of Cakes
Our platform showcases a variety of cakes in different categories. From classic vanilla and chocolate to exotic flavors, we have something for everyone.
Custom Cake Ordering
Want something unique? We offer custom cake ordering options where you can specify the cake’s flavor, size, shape, and even mechanical cake designs.
Multiple Payment Options
We offer multiple payment methods for your convenience, including credit card payments and cash on delivery.
Email Confirmation
Once your transaction is successful, you’ll receive an email confirmation along with a copy of your shopping receipt.
Swiggy Integration
For those who prefer using Swiggy for food delivery, we’ve got you covered. Our cake ordering system is integrated with Swiggy, making your cake delivery even more convenient.
Advantages
- Automates the process of selling cakes online, making it easier for both buyers and sellers.
- Accepts credit card payments, offering a secure transaction process.
- Provides email confirmation upon successful payment, keeping you informed every step of the way.
Disadvantages
- Currently, our system does not keep track of stock, so make sure to order your favorite cakes before they run out!
Conclusion
Our Online Cake Delivery System is designed to make your cake shopping experience as smooth as possible. With features like custom cake ordering and multiple payment options, we aim to be your go-to platform for all your cake needs.
Sample Code
First, install Flask and Flask-SQLAlchemy:
pip install Flask Flask-SQLAlchemy
from flask import Flask, render_template, request, redirect, url_for
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///cakes.db'
db = SQLAlchemy(app)
class Cake(db.Model):
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(50), nullable=False)
price = db.Column(db.Float, nullable=False)
@app.route('/')
def index():
cakes = Cake.query.all()
return render_template('index.html', cakes=cakes)
@app.route('/order/<int:cake_id>', methods=['POST'])
def order_cake(cake_id):
cake = Cake.query.get_or_404(cake_id)
# Here you would handle the ordering logic, e.g., payment, email confirmation, etc.
return redirect(url_for('index'))
if __name__ == '__main__':
db.create_all()
app.run(debug=True)
For the HTML templates, create a folder named templates
in the same directory as your Flask application and add an index.html
file:
<!DOCTYPE html>
<html>
<head>
<title>Online Cake Shop</title>
</head>
<body>
<h1>Welcome to the Online Cake Shop</h1>
<ul>
{% for cake in cakes %}
<li>
{{ cake.name }} - ${{ cake.price }}
<form action="{{ url_for('order_cake', cake_id=cake.id) }}" method="post">
<button type="submit">Order Now</button>
</form>
</li>
{% endfor %}
</ul>
</body>
</html>
This is a very basic example. In a real-world application, you’d likely want to add more features like user authentication, payment gateway integration, email confirmations, and so on.
To run the code, save the Python code in a file (e.g., app.py
), and the HTML in a templates
folder. Run app.py
, and you should be able to see the cake list at http://127.0.0.1:5000/
.
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Follow On YoutubeSubscribed? Click on Confirm
Download Online Cake Delivery System PDF
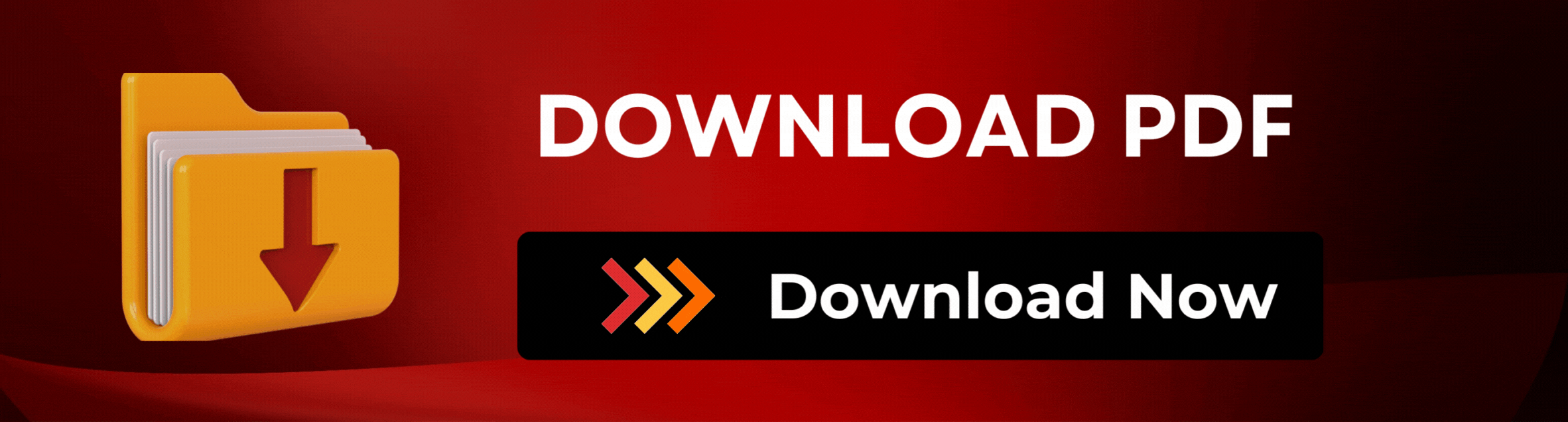