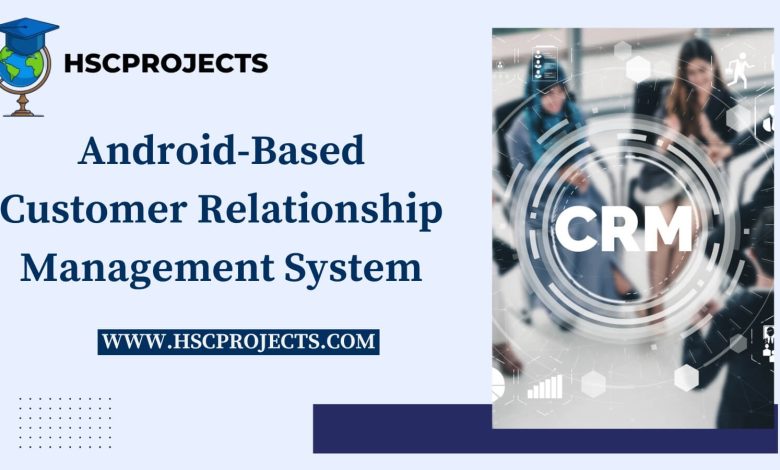
Android-Based Customer Relationship Management System
Introduction
Customer Relationship Management (CRM) has always been at the core of successful businesses. However, the advent of technology has significantly improved how companies manage their interactions with current and potential customers. This article delves into the intricacies of Android-based Customer Relationship Management Systems, a technology that combines the power of mobile computing with traditional CRM functionalities.
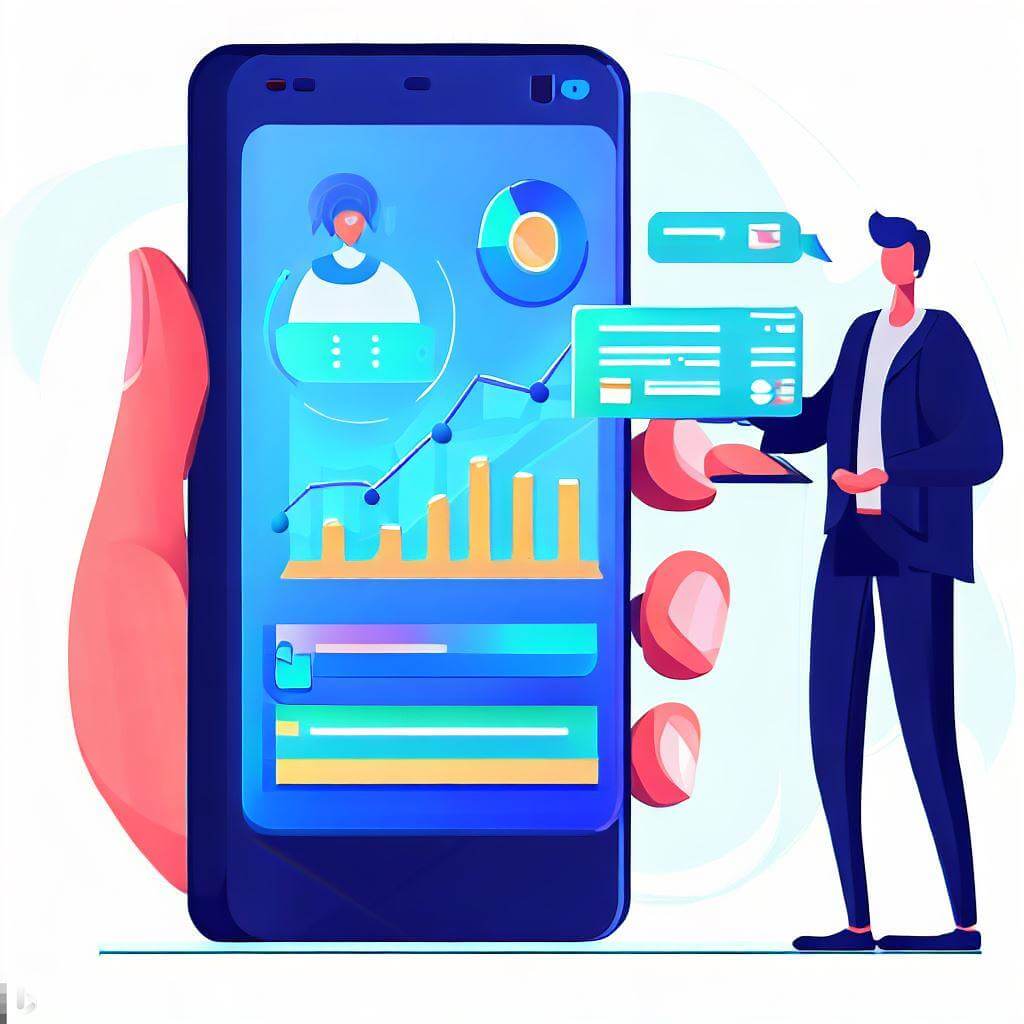
System Overview
The Android-based Customer Relationship Management System is designed to streamline various business processes, from lead generation to sales conversion and customer service. Unlike traditional CRM software, this system is mobile, allowing for real-time updates and greater flexibility for employees on the go.
Key Features
- Employee Onboarding: Admins can easily add new employees, providing them with a unique customer relationship number and password to access the system.
- Dashboard Insights: Upon login, employees are greeted with a dashboard that displays key metrics, including the number of leads converted and pending, as well as profit and loss figures.
- Lead Management: Employees can register new leads by entering customer details into the customer information management system.
- Meeting Scheduling: The system allows employees to schedule meetings with customers, complete with topics and descriptions.
- Communication: Features for sending SMS and emails to customers are integrated into the system.
Advantages
- Enhanced Sales Management: The system offers a comprehensive view of each employee’s sales performance, making it easier to track and meet targets.
- Efficient Customer Engagement: With the mobile app, employees can engage with customers more effectively, whether they’re in the office or out in the field.
- Automated Processes: The system automates many routine tasks, allowing employees to focus on more strategic customer relationship management project topics.
Limitations
- Device Dependency: The system is designed for Android, so users without an Android device cannot access the application.
- Internet Requirement: A stable internet connection is necessary for the system to function optimally.
Conclusion
Android-based Customer Relationship Management Systems offer a versatile and effective solution for modern businesses. By adopting this technology, companies can not only improve their sales processes but also enhance customer satisfaction, thereby achieving long-term success.
Sample Code
MainActivity.java
package com.example.crmapp;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
EditText customerName, customerEmail, customerPhone;
Button saveButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
customerName = findViewById(R.id.customerName);
customerEmail = findViewById(R.id.customerEmail);
customerPhone = findViewById(R.id.customerPhone);
saveButton = findViewById(R.id.saveButton);
saveButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String name = customerName.getText().toString();
String email = customerEmail.getText().toString();
String phone = customerPhone.getText().toString();
// Here you would typically send these details to your backend server
// For demonstration, we'll just show a toast message
Toast.makeText(MainActivity.this, "Customer details saved: " + name + ", " + email + ", " + phone, Toast.LENGTH_LONG).show();
}
});
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/customerName"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Customer Name"/>
<EditText
android:id="@+id/customerEmail"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/customerName"
android:hint="Customer Email"/>
<EditText
android:id="@+id/customerPhone"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/customerEmail"
android:hint="Customer Phone"/>
<Button
android:id="@+id/saveButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/customerPhone"
android:text="Save"/>
</RelativeLayout>
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Subscribed? Click on Confirm
Download Android-Based Customer Relationship Management System PDF
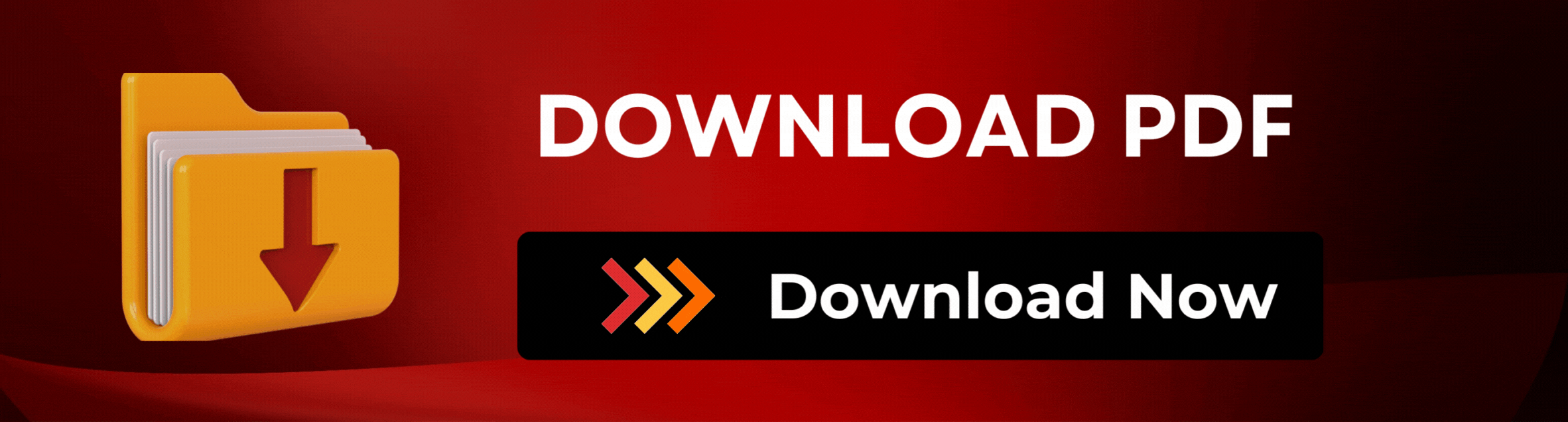