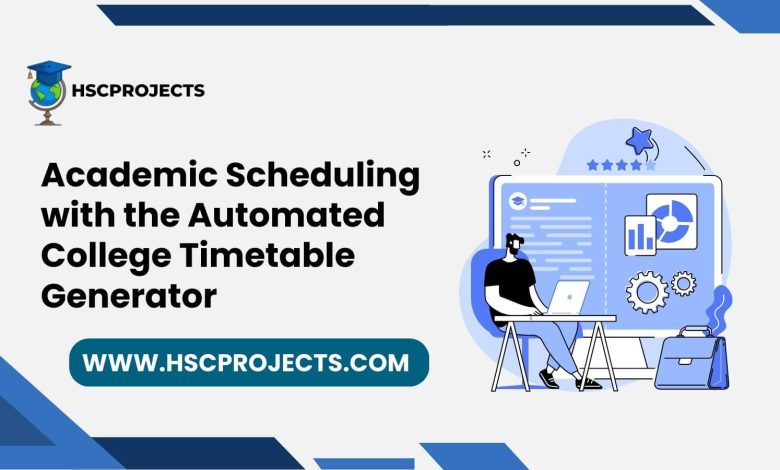
Academic Scheduling with the Automated College Timetable Generator
Introduction
Managing a college timetable is a complex task that involves accommodating various courses, subjects, and faculty schedules. Traditional methods often require manual adjustments and can be time-consuming. Enter the Automated College Timetable Generator, a game-changing solution that employs genetic algorithms to create efficient and conflict-free timetables.

How It Works
The Automated Timetable Generator uses a unique object-oriented approach. The primary object is the ‘Timetable,’ which contains multiple ‘Classroom’ objects. Each Classroom object, in turn, contains ‘Week’ objects, which are further divided into ‘Days.’ Each Day contains ‘Timeslots,’ where specific details like the subject, faculty, and student group are stored.
The system assigns a ‘fitness score’ to each timetable, representing the number of scheduling conflicts or overlaps. The genetic algorithm iteratively refines the timetable to minimize this score, ensuring an optimized schedule.
Key Features
- Genetic Algorithm: Utilizes evolutionary techniques to optimize the timetable.
- Fitness Score: Quantifies the efficiency of the timetable, aiming for the lowest score possible.
- Object-Oriented Design: Makes the system easily extendable and adaptable.
- Constraint Checking: Built-in checks for faculty availability, classroom occupancy, and other logistical constraints.
Advantages
- No Time Clashes: Faculty can focus on teaching rather than juggling schedules.
- Efficient Resource Allocation: Makes optimal use of available classrooms and time slots.
- Time-Saving: Frees up administrative resources, allowing them to focus on other important tasks.
Disadvantages
- Minor Formatting Required: The generated timetable may require some manual adjustments for specific preferences or constraints.
Conclusion
The Automated College Timetable Generator is a revolutionary tool that brings efficiency and accuracy to academic scheduling. By leveraging advanced algorithms and an intuitive object-oriented design, it significantly reduces the manual effort involved in timetable management.
Sample Code
First, you’ll need to install the deap
library for genetic algorithms:
pip install deap
from deap import base, creator, tools, algorithms
import random
# Define the fitness function
def fitness(individual):
score = 0
for i in range(len(individual) - 1):
if individual[i] == individual[i + 1]:
score += 1
return score,
# Create classes for the problem
creator.create("FitnessMin", base.Fitness, weights=(-1.0,))
creator.create("Individual", list, fitness=creator.FitnessMin)
toolbox = base.Toolbox()
# Create the 'individual' and 'population'
toolbox.register("attr_int", random.randint, 1, 10)
toolbox.register("individual", tools.initRepeat, creator.Individual, toolbox.attr_int, 10)
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
# Register genetic operators
toolbox.register("mate", tools.cxTwoPoint)
toolbox.register("mutate", tools.mutUniformInt, low=1, up=10, indpb=0.2)
toolbox.register("select", tools.selTournament, tournsize=3)
toolbox.register("evaluate", fitness)
# Create a population
population = toolbox.population(n=50)
# Run the genetic algorithm
algorithms.eaSimple(population, toolbox, cxpb=0.5, mutpb=0.2, ngen=10, stats=None, halloffame=None, verbose=True)
# Extract and print the best timetable from the final population
best_individual = tools.selBest(population, k=1)[0]
print("Best timetable:", best_individual)
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Subscribed? Click on Confirm
Download Academic Scheduling with the Automated College Timetable Generator PDF
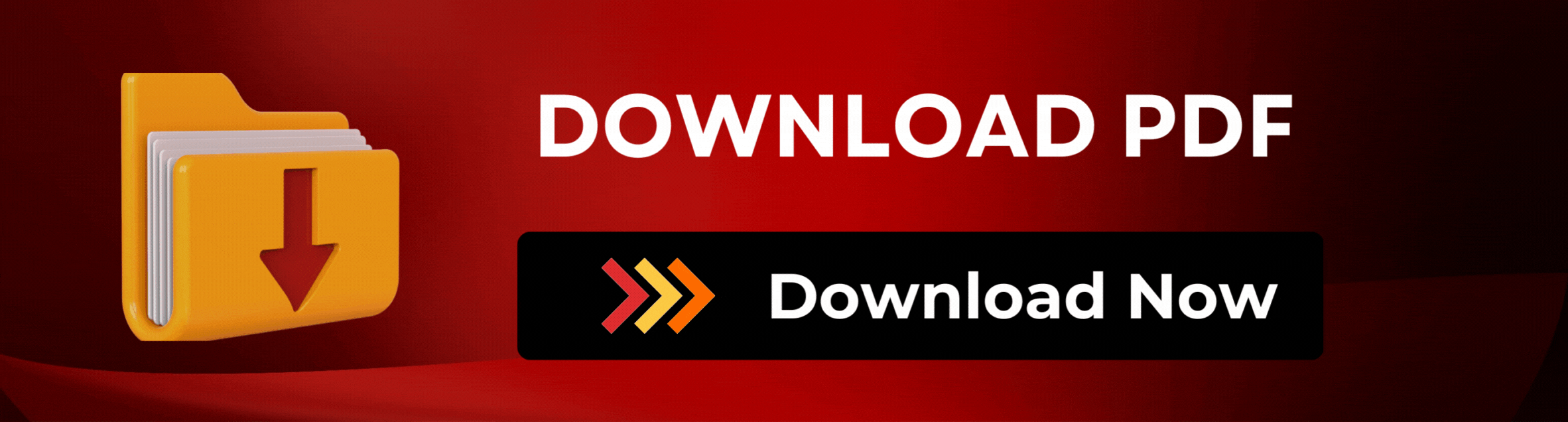