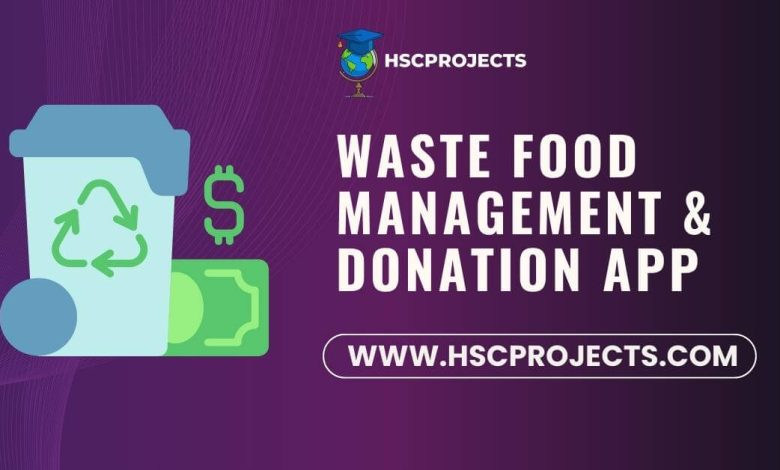
Waste Food Management & Donation App
The Global Food Waste Dilemma
It’s a staggering reality: while nearly a third of all food produced for human consumption goes to waste, amounting to 1.3 billion tons annually, a significant portion of the global population grapples with food shortages. The data from the Food and Agriculture Organization underscores the urgency of addressing this imbalance. But how can we bridge the gap between excess food and those who desperately need it?
Introducing the Food Waste Management System
Our innovative food waste management system, available as an Android application, offers a solution. Designed to connect restaurants and hotels with leftover food to NGOs dedicated to combating hunger, this food donation app ensures that excess food finds its way to those in need rather than ending up in landfills.
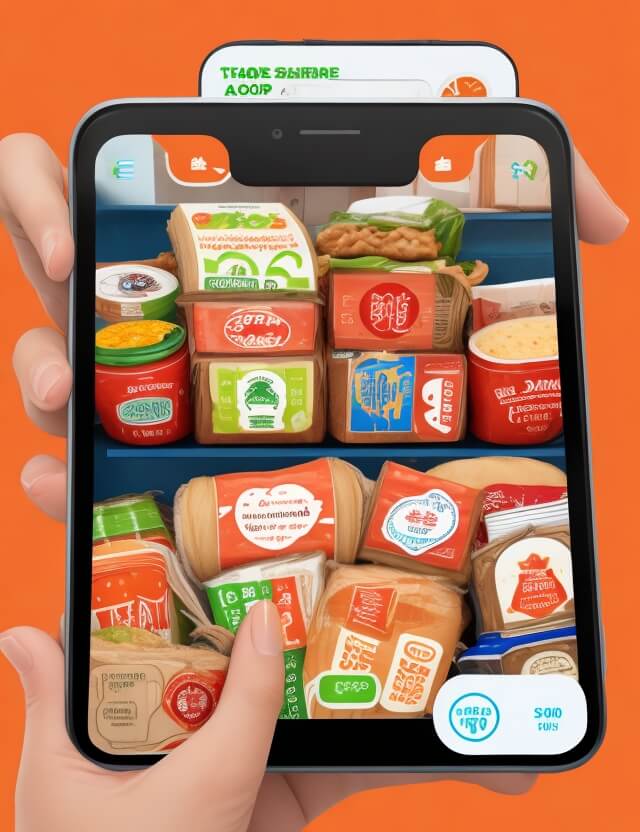
How It Works:
- NGO Requests: NGOs can raise a request for excess food through the app.
- Restaurant Approval: Participating restaurants receive these requests and can approve them based on their leftover food availability.
- Food Collection: Once approved, NGOs can collect the food for distribution among the needy.
- Tracking and History: The system maintains a record of all transactions, allowing for transparency and accountability.
Advantages of the Food Donation App:
- Dual Benefit: Restaurants can reduce food wastage, and the underprivileged receive nourishment.
- Wastage Tracking: Restaurants can monitor and manage their food wastage more effectively.
- Community Impact: Users play a pivotal role in reducing food wastage and supporting the needy.
Potential Limitations:
While the food wastage management system project is transformative, it’s essential to be aware of potential challenges:
- Data Accuracy: Incorrect inputs can impact the system’s efficiency.
- Connectivity Dependence: A stable internet connection is crucial for uninterrupted operations.
- Server Reliability: The system relies on server uptime, making it vulnerable to single-point failures.
Conclusion:
The food waste management project is not just an app; it’s a movement. By bridging the gap between excess food and those in need, we’re taking a significant step towards a world where food wastage is minimized, and every individual has access to a nutritious meal.
Requirements:
- Flask
- Flask-SQLAlchemy
You can install them using pip:
pip install Flask Flask-SQLAlchemy
Sample Code:
from flask import Flask, render_template, request, redirect, url_for, flash
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///food_donation.db'
app.config['SECRET_KEY'] = 'mysecretkey'
db = SQLAlchemy(app)
class Restaurant(db.Model):
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(80), unique=True, nullable=False)
leftover_food = db.Column(db.Boolean, default=False)
requests = db.relationship('NGO', backref='restaurant', lazy=True)
class NGO(db.Model):
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(80), unique=True, nullable=False)
request_status = db.Column(db.String(80), default='Pending')
restaurant_id = db.Column(db.Integer, db.ForeignKey('restaurant.id'), nullable=True)
@app.route('/')
def index():
restaurants = Restaurant.query.all()
ngos = NGO.query.all()
return render_template('index.html', restaurants=restaurants, ngos=ngos)
@app.route('/request_food/<int:restaurant_id>', methods=['POST'])
def request_food(restaurant_id):
ngo_name = request.form.get('ngo_name')
ngo = NGO(name=ngo_name, restaurant_id=restaurant_id)
db.session.add(ngo)
db.session.commit()
flash(f"Request sent by {ngo_name} to the restaurant!", "success")
return redirect(url_for('index'))
@app.route('/approve_request/<int:ngo_id>', methods=['POST'])
def approve_request(ngo_id):
ngo = NGO.query.get(ngo_id)
ngo.request_status = 'Approved'
db.session.commit()
flash(f"Request by {ngo.name} has been approved!", "success")
return redirect(url_for('index'))
if __name__ == '__main__':
db.create_all()
app.run(debug=True)
HTML Template (templates/index.html
):
<!DOCTYPE html>
<html>
<head>
<title>Food Donation App</title>
</head>
<body>
<h1>Welcome to the Food Donation App</h1>
<h2>Restaurants</h2>
<table>
<thead>
<tr>
<th>Name</th>
<th>Leftover Food</th>
<th>Action</th>
</tr>
</thead>
<tbody>
{% for restaurant in restaurants %}
<tr>
<td>{{ restaurant.name }}</td>
<td>{{ restaurant.leftover_food }}</td>
<td>
<form action="{{ url_for('request_food', restaurant_id=restaurant.id) }}" method="post">
<input type="text" name="ngo_name" placeholder="Enter NGO Name">
<button type="submit">Request Food</button>
</form>
</td>
</tr>
{% endfor %}
</tbody>
</table>
<h2>NGOs</h2>
<table>
<thead>
<tr>
<th>Name</th>
<th>Request Status</th>
<th>Action</th>
</tr>
</thead>
<tbody>
{% for ngo in ngos %}
<tr>
<td>{{ ngo.name }}</td>
<td>{{ ngo.request_status }}</td>
<td>
{% if ngo.request_status == 'Pending' %}
<form action="{{ url_for('approve_request', ngo_id=ngo.id) }}" method="post">
<button type="submit">Approve</button>
</form>
{% endif %}
</td>
</tr>
{% endfor %}
</tbody>
</table>
</body>
</html>
In order to download the PDF, You must follow on Youtube. Once done, Click on Submit
Subscribed? Click on Confirm
Download Waste Food Management & Donation App PDF
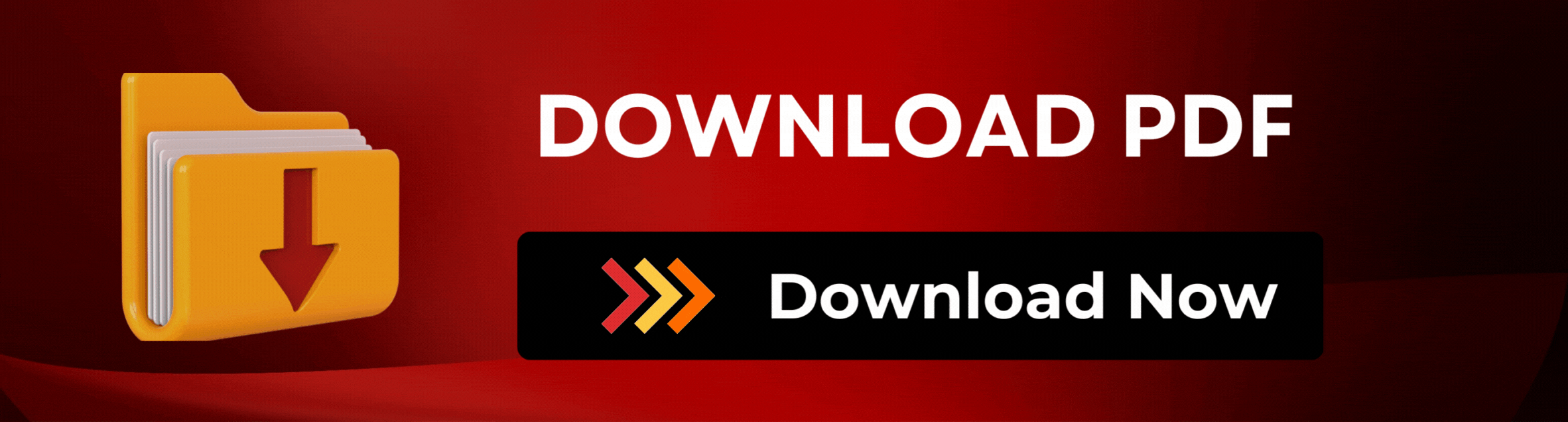